Bitwise Operation
A bitwise operation is a technique used in computer programming to manipulate individual bits of binary numbers. It involves performing logical operations on corresponding bits of two binary numbers.
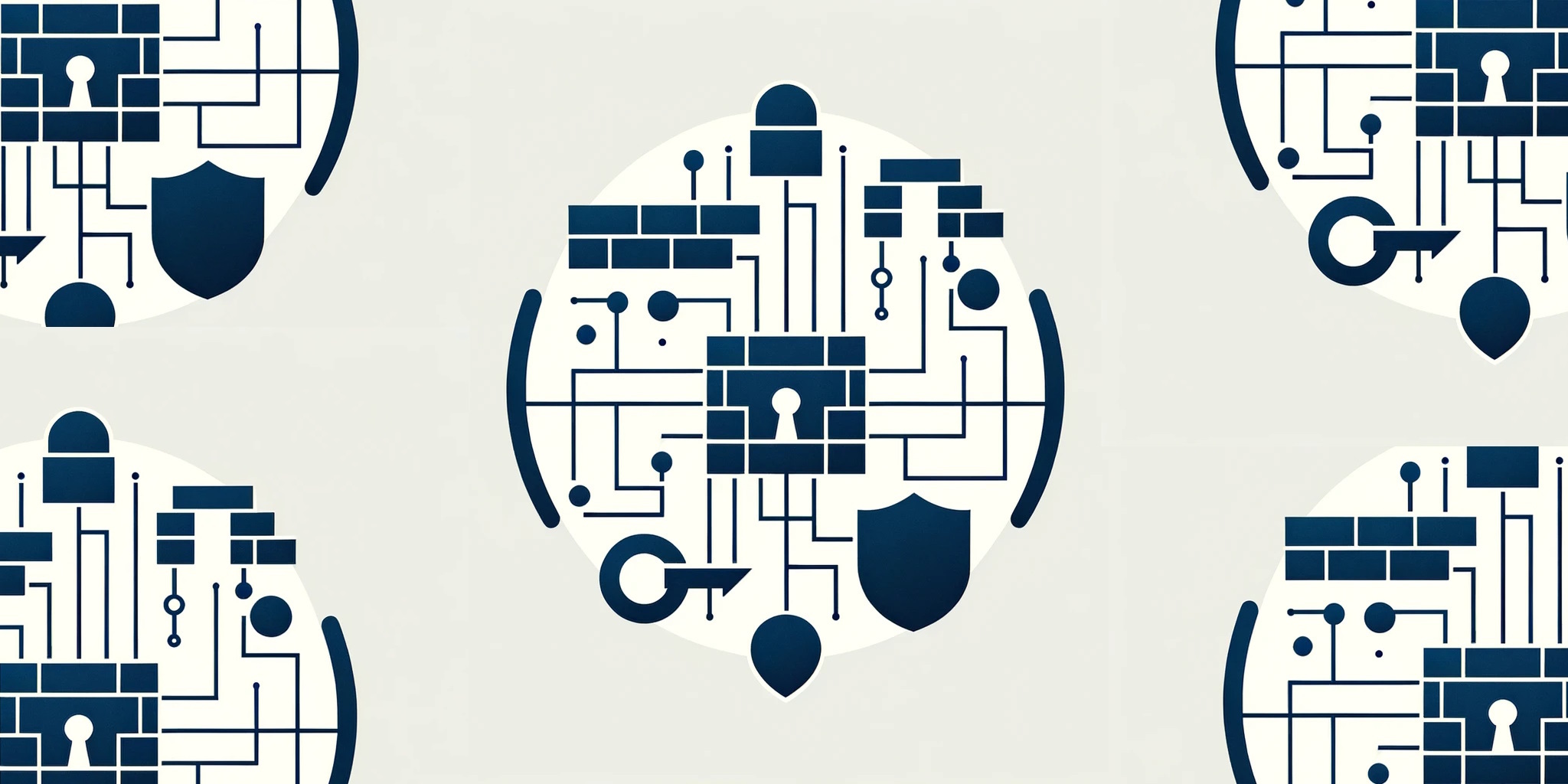
How Bitwise Operations Work
Bitwise operations work by comparing the bits of two binary numbers and producing a new binary number as a result. Here are the most common bitwise operations:
- AND Operation:
The AND operation compares each bit of two numbers. If both bits are 1, the result is 1; otherwise, it's 0. It can be represented using the "&" symbol.
Example:
```
10101010
& 11001100
10001000
```
In this example, the result of the AND operation is 10001000.
- OR Operation:
The OR operation compares each bit of two numbers. If at least one bit is 1, the result is 1; if both bits are 0, the result is 0. It can be represented using the "|" symbol.
Example:
```
10101010
| 11001100
11101110
```
In this example, the result of the OR operation is 11101110.
- XOR Operation:
The XOR operation compares each bit of two numbers. If the bits are different, the result is 1; if they are the same, the result is 0. It can be represented using the "^" symbol.
Example:
```
10101010
^ 11001100
01100110
```
In this example, the result of the XOR operation is 01100110.
- NOT Operation:
The NOT operation flips each bit of a number, changing 1 to 0 and 0 to 1. It can be represented using the "~" symbol.
Example:
```
~ 10101010
01010101
```
In this example, the result of the NOT operation is 01010101.
Practical Uses of Bitwise Operations
Bitwise operations have various practical uses in computer programming. Some common applications include:
- Masking:
Masking involves setting, clearing, or toggling specific bits of a binary number to control certain hardware settings or flags in a program. By using bitwise operations, programmers can manipulate specific bits without affecting the other bits of the number.
Example:
// Set the 4th and 5th bits to 1, while keeping other bits unchanged
int number = 0b00110011;
int mask = 0b00011000;
int result = number | mask; // result = 0b00111011
- Encryption:
Bitwise operations can be used in encryption algorithms to obfuscate information for security purposes. By manipulating bits using bitwise operations, data can be scrambled or encoded in a way that makes it difficult to understand without the proper decoding process.
Example:
// XOR encryption
int data = 0b11001100;
int key = 0b10101010;
int encryptedData = data ^ key; // encryptedData = 0b01100110
- Performance Optimization:
In cases where speed is crucial, bitwise operations can be used to perform arithmetic operations more efficiently. Bitwise operations require fewer computational resources compared to traditional arithmetic operations, making them useful in performance-critical scenarios.
Example:
// Multiplication by powers of 2 using left shift
int number = 5;
int result = number << 3; // result = 5 * 2^3 = 40
Prevention Tips
When using bitwise operations for security purposes, it is important to ensure that the algorithms are implemented securely to prevent vulnerabilities. Here are some prevention tips:
- Use well-known and tested cryptographic algorithms rather than attempting to create custom bitwise operations for encryption.
- Regularly update and patch the encryption algorithms to address any discovered vulnerabilities.
Remember that bitwise operations can be highly efficient and useful, but it is essential to understand their limitations and ensure their secure implementation when dealing with sensitive information.