Dynamic Memory Allocation
Dynamic memory allocation is a programming concept that allows the allocation of memory for variables at runtime, rather than at compile time. It enables a program to allocate memory for data whose size is not known until the program is executing.
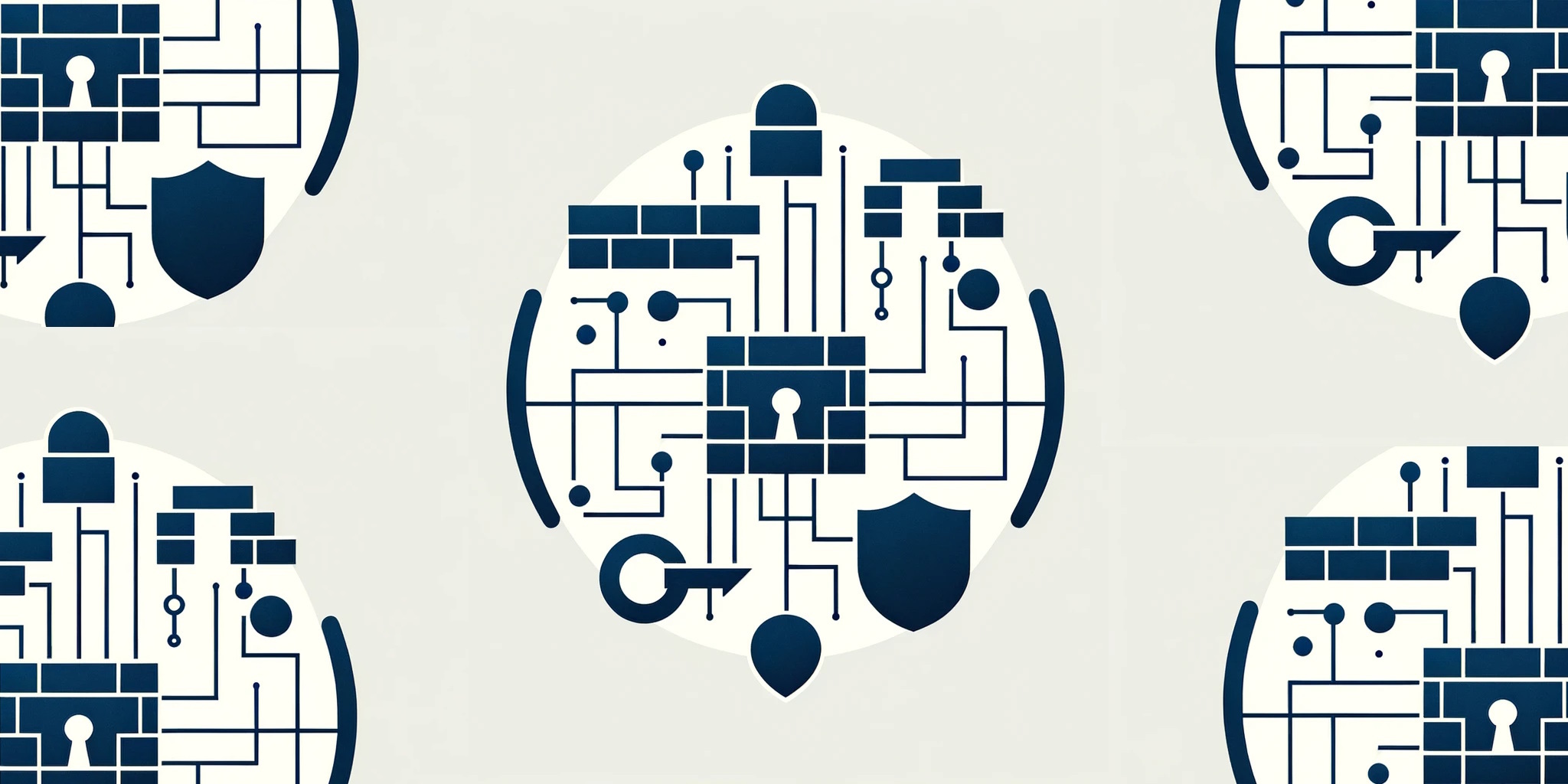
How Dynamic Memory Allocation Works
When a program needs memory for variables whose size cannot be determined at compile time, it requests memory from the system dynamically during runtime. This is commonly done using functions specific to the programming language being used. Here are some examples:
- C and C++: In C and C++, the
malloc
function is used to dynamically allocate memory. It returns a pointer to the allocated memory block.
- C++: In C++, the
new
operator can be used to allocate memory for objects. It not only allocates memory but also calls the object's constructor.
- Python: In Python, memory allocation is handled automatically by the Python interpreter. Memory is allocated when objects are created and deallocated when they are no longer in use. The
alloc
function is provided in Python, but it is uncommon to use it in regular programming.
The memory allocated dynamically is done on the heap, a region of memory separate from the stack where static memory is allocated. The heap is used for storing data structures such as arrays, linked lists, and objects. Once the dynamically allocated memory is no longer needed, it must be explicitly deallocated to prevent memory leaks, which can result in the wastage of memory resources. In C and C++, the free
function is used to deallocate memory, while in C++, the delete
operator is used to both deallocate memory and call the destructor of the object.
Benefits of Dynamic Memory Allocation
Dynamic memory allocation provides several benefits in programming:
- Flexibility: It allows programs to allocate memory based on the actual requirements at runtime. This is particularly useful when dealing with data structures that need to grow or shrink dynamically.
- Efficiency: By allocating memory as needed, programs can make optimal use of system resources, avoiding unnecessary memory consumption.
- Reusability: Dynamically allocated memory can be reused for different purposes within the program, enhancing code modularity and flexibility.
- Complex data structures: It enables the creation of complex data structures such as linked lists, trees, and graphs, which may vary in size and structure during program execution.
Best Practices for Dynamic Memory Allocation
To effectively use dynamic memory allocation, it is important to follow these best practices:
- Always free dynamically allocated memory: After dynamically allocating memory, it is crucial to release it when it is no longer needed. Failing to do so leads to memory leaks, where the program consumes memory without releasing it back to the system.
- Avoid accessing or modifying deallocated memory: Once memory is deallocated, any attempt to access or modify it leads to behavior and can result in program crashes, data corruption, or security vulnerabilities.
- Use automatic memory management: Modern programming languages and libraries, such as Java, Python, and C#, offer automatic memory management through techniques such as garbage collection. Using these languages can help eliminate many of the challenges and pitfalls associated with manual memory management.
Related Terms
- Memory Leak: When a program fails to release memory it has allocated, leading to the wastage of memory resources.
- Buffer Overflow: A security vulnerability that occurs when a program writes more data to a block of memory, or buffer, than it can hold, potentially leading to a security breach.
Dynamic memory allocation is an essential concept in programming that enables the allocation and deallocation of memory at runtime. By understanding how it works and following best practices, developers can effectively manage memory resources, optimize program performance, and ensure the stability and security of their applications.