Mutex (Mutual Exclusion) Definition
A mutex, short for "mutual exclusion," is a programming construct that ensures only one process at a time can access a particular resource or piece of code. It is commonly used in software development to prevent multiple threads from simultaneously modifying shared data, which can lead to unpredictable and erroneous behavior in a program.
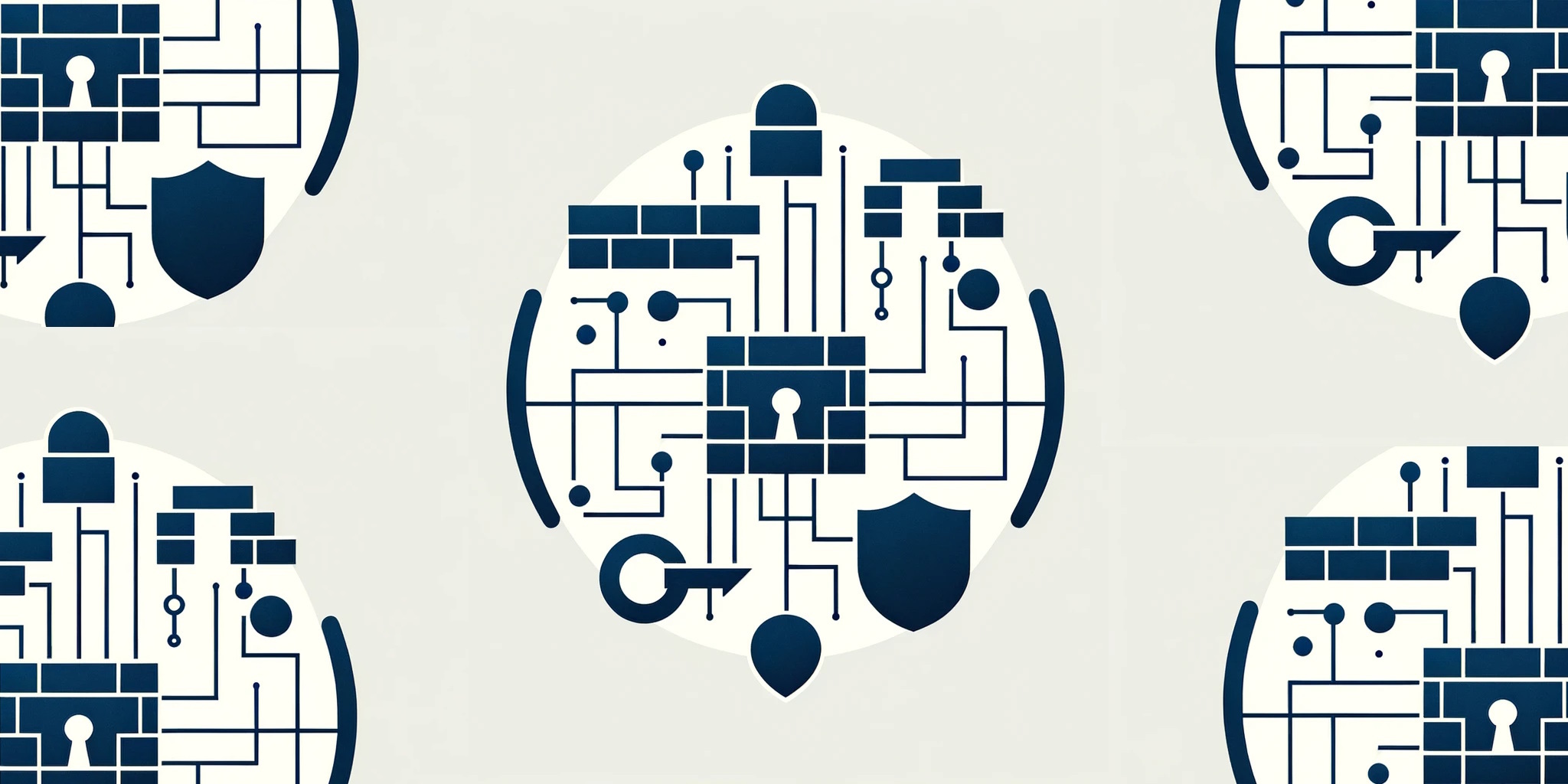
Key Concepts
- Mutexes provide a way to synchronize access to shared resources in multi-threaded applications by allowing one thread to gain exclusive access while blocking others.
- They are often implemented using hardware support or operating system primitives, such as atomic operations or system calls.
- Mutexes can be created and used within a single process or shared between multiple processes in a concurrent system.
How Mutex Works
When multiple threads or processes need access to a shared resource, they must first acquire the associated mutex. If another thread has already acquired the mutex, the requesting thread will be blocked until the mutex is released. Once the resource is no longer needed, the thread releases the mutex, allowing other threads to acquire it.
To ensure the correct usage of mutexes, the following guidelines should be followed:
- Always acquire the mutex before accessing the shared resource.
- Release the mutex after finishing the operations on the shared resource.
- Avoid holding the mutex for a longer time to minimize contention and improve performance.
- Acquire and release mutexes in a consistent order to avoid deadlocks.
Examples
Example 1:
Consider a banking application that allows multiple threads to withdraw money from the same account. To prevent race conditions and ensure the account balance is updated correctly, a mutex can be used:
```python
class BankAccount:
def init(self, balance):
self.balance = balance
self.mutex = Mutex()
def withdraw(self, amount):
self.mutex.acquire()
if self.balance >= amount:
self.balance -= amount
print("Withdrawal successful. Remaining balance:", self.balance)
else:
print("Insufficient balance.")
self.mutex.release()
account = BankAccount(1000)
```
In this example, the producer
function acquires the mutex, enqueues an item in the shared queue
, and then releases the mutex. Similarly, the consumer
function acquires the mutex, checks if the queue
is not empty, dequeues an item, processes it, and releases the mutex.
Additional Notes
- Mutexes are a fundamental synchronization primitive in concurrent programming and are widely used in various applications, including operating systems, database management systems, and networking protocols.
- While mutexes provide a simple and effective way to manage shared resources safely, their incorrect usage can lead to issues such as deadlocks and priority inversion.
- Deadlocks occur when multiple threads are waiting indefinitely for each other to release the mutexes they hold, causing the system to become unresponsive.
- Priority inversion can occur when a low-priority thread holding a mutex prevents a high-priority thread from executing, leading to priority inversion and potential performance degradation.
Related Terms
- Semaphore: Another synchronization mechanism used for managing access to shared resources in a multi-threaded environment.
- Race Condition: A situation where the outcome of a program depends on the relative timing of multiple threads or processes accessing shared data.
- Concurrency: The ability of different parts or units of a program to be executed out-of-order or in partial order without affecting the final outcome.