Structured Programming Definition
Structured programming is a programming paradigm that advocates for the use of structured control flow constructs, such as sequences, selection, and iteration, to create clear, understandable, and maintainable code. It focuses on breaking down a program into smaller, manageable, and reusable pieces to improve readability, support modular design, and reduce error-prone coding practices.
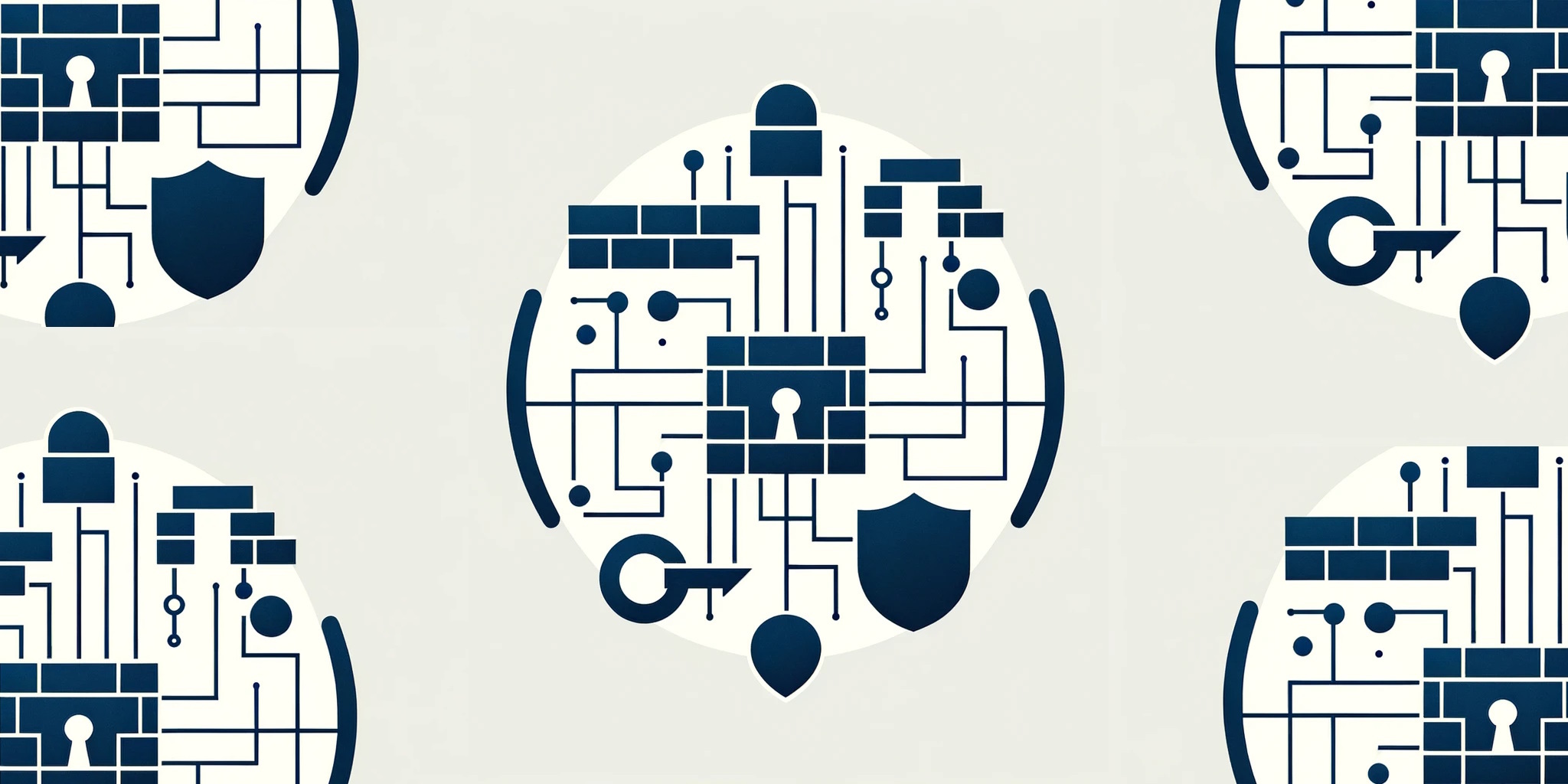
Structured programming promotes a disciplined approach to software development and is characterized by its emphasis on the following principles:
- Structured Control Flow: The foundation of structured programming lies in the use of well-defined control flow constructs. These include:
- Sequential Execution: The program is executed line by line, in the order that statements appear. This improves code readability and enables step-by-step execution for easy debugging.
- Selection: This includes if-then-else statements, switch-case statements, or other conditional branching constructs to choose between different actions based on certain conditions. This enables the program to make decisions and execute different code paths accordingly.
- Iteration: The use of loops (e.g., for, while, do-while) allows for the repeated execution of a block of code. This is particularly useful when dealing with repetitive tasks or data processing.
These structured control flow constructs help in breaking down complex problems into smaller, more manageable parts, leading to improved code understanding, maintainability, and bug detection.
Prevention Tips
When practicing structured programming, consider the following tips:
- Use Modularization: Break down your program into smaller, distinct, and reusable modules or functions. Each module should have a specific purpose and encapsulate related functionality. This promotes code reuse, simplifies maintenance, and improves overall program comprehension.
- Avoid Goto Statements: Minimize the use of unstructured control flow constructs like the
goto
statement, which can lead to spaghetti code. Spaghetti code refers to code that is difficult to read and understand due to its unorganized and erratic jumps. By avoiding goto
statements, you can maintain a clear and linear control flow in your program.
- Adopt Design Patterns: Design patterns are reusable solutions to common software design problems. By implementing well-established design patterns, you can promote code reuse, maintainability, and scalability. Some commonly used design patterns in structured programming include the module pattern, factory pattern, and observer pattern.
Using structured programming principles not only results in more manageable and less error-prone code but also helps in improving software quality and reducing development time.
Examples
Let's consider a few examples to illustrate the application of structured programming:
Calculating the Average of Numbers: Suppose you have a list of numbers and you want to calculate their average. Using structured programming, you can break down the task into smaller steps:
- Iterate through the list and sum all the numbers.
- Divide the sum by the total number of elements to calculate the average.
- Return the average.
Sorting an Array: To sort an array in ascending order, you can use a structured approach:
- Iterate through the array elements.
- Compare adjacent elements and swap them if they are out of order.
- Repeat the process until the array is sorted.
By breaking down complex tasks into smaller steps and utilizing structured control flow constructs, you can write code that is easier to understand, maintain, and test.
Related Terms
- Procedural Programming: A type of programming paradigm that follows a top-down approach, emphasizing procedures or functions. Procedural programming, like structured programming, focuses on the use of modular code and structured control flow.
- Object-Oriented Programming (OOP): A programming paradigm based on the concept of objects, which can contain data and code that manipulates the data. OOP provides encapsulation, inheritance, and polymorphism as mechanisms to structure and organize code.
- Control Flow: The order in which the computer executes statements in a piece of code. Control flow is determined by structured control flow constructs, such as conditionals and loops, which dictate the flow of program execution.