Try-Catch Blocks Definition
In programming, a try-catch block is a mechanism for handling errors or exceptions that might occur during the execution of a program. It provides a structured way to handle and recover from errors, ensuring that the program does not crash abruptly. A try-catch block consists of a "try" block where the code that might cause an error is enclosed, and a "catch" block that handles the specific type of error if it occurs.
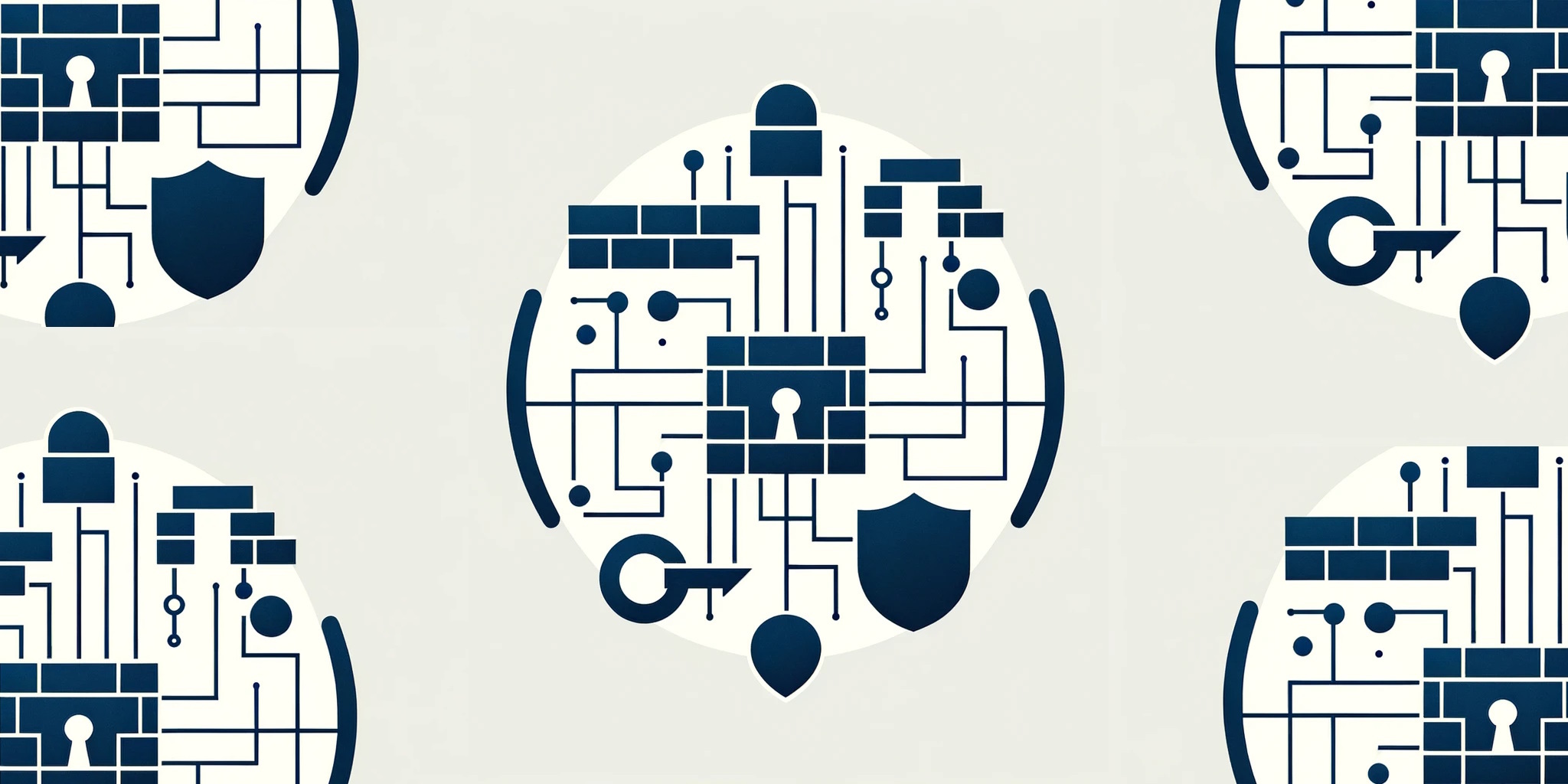
How Try-Catch Blocks Work
When a try-catch block is implemented, the following steps occur:
- Within the try block, the code that could potentially cause an error is enclosed. This can include statements, functions, or operations that may result in exceptions.
- If an error or exception occurs within the try block, the control of the program is transferred to the catch block. The catch block contains the code that handles the error.
- The catch block specifies the type of error it can handle. When an error matching the specified type occurs, the catch block is executed to handle the exception.
- The catch block contains the necessary code to handle the error. This can include logging the error, displaying an error message to the user, or performing alternative actions to recover from the error.
- After the catch block is executed, the program continues executing the code that follows it, allowing the program to gracefully recover from the error and continue its execution flow.
Prevention Tips
Try-catch blocks are an essential tool for creating robust programs that can handle unexpected errors. Here are some tips for effectively using try-catch blocks:
- Identify potential error points: Identify the areas in your code where errors are likely to occur. This could be when accessing external resources, manipulating data, or performing complex operations.
- Enclose error-prone code: Enclose the code that may cause errors within a try block. By doing this, you ensure that any exceptions thrown by the enclosed code will be caught and handled appropriately.
- Specify specific exception types: In the catch block, specify the type of exception you expect to handle. This allows you to have different catch blocks for handling different types of exceptions, providing more specific error handling and recovery options.
- Handle exceptions gracefully: In the catch block, handle the exception gracefully. This can include logging the error information for debugging purposes, displaying user-friendly error messages, or executing alternative actions to recover from the error.
- Consider multiple catch blocks: If your code can potentially throw different types of exceptions, consider using multiple catch blocks to handle each exception separately. This allows for more granular error handling and recovery.
- Add a finally block (optional): You can also include a finally block after the catch block to specify code that should be executed regardless of whether an exception occurred or not. This is useful for performing cleanup operations or releasing resources.
By following these prevention tips, you can anticipate and handle potential errors in your code effectively, making your programs more robust and preventing unexpected terminations.
Related Terms
- Exception Handling: Exception handling is the process of responding to the occurrence of exceptions. It encompasses various techniques and mechanisms, including try-catch blocks, to deal with errors in programs.
- Error Handling: Error handling refers to the techniques and practices used to manage errors in code. Try-catch blocks and other error prevention methods are essential components of error handling strategies.