Typecasting
Typecasting Definition
Typecasting refers to the process of converting a variable from one data type to another in computer programming. It allows the programmer to interpret and manipulate data in different ways, depending on the desired outcome. Typecasting is a common practice in many programming languages and is used to ensure compatibility and consistency in data operations.
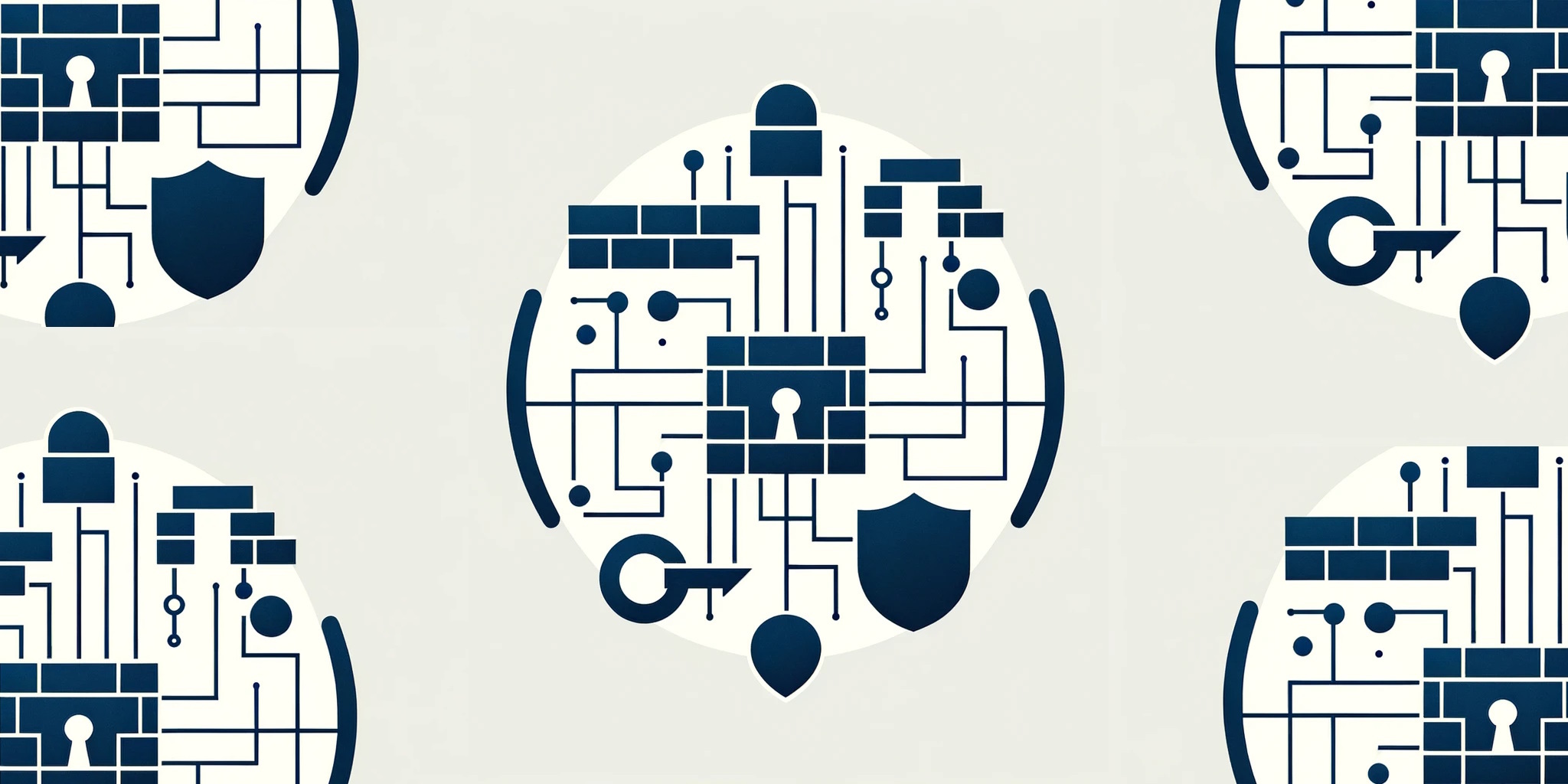
How Typecasting Works
In programming, variables have specific data types that determine the kind of data they can hold and the operations that can be performed on them. When typecasting, a programmer explicitly instructs the compiler or interpreter to treat a variable as a different data type, overriding its original type. This can be done in two ways:
Implicit Typecasting:
- In some cases, the compiler or interpreter automatically converts a variable from one type to another if it is required for an operation. This is known as implicit typecasting or coercion.
- For example, if you divide an integer by a floating-point number, the compiler may automatically convert the integer to a floating-point number before performing the operation.
- Implicit typecasting can be convenient, but it may also lead to unexpected results if not handled carefully.
Explicit Typecasting:
- Explicit typecasting, also known as type conversion, involves the programmer explicitly specifying the desired data type for a variable.
- This is done by using type conversion operators or functions provided by the programming language, such as
int()
, float()
, or str()
.
- For example, if you have a floating-point number and you want to use it as an integer, you can use the
int()
function to convert it explicitly.
Examples and Use Cases
Typecasting is used in various scenarios to handle different data types and perform specific operations. Here are some examples:
Integer to Float:
- Typecasting an integer to a float allows for more precise calculations and the inclusion of fractional values.
- For example, if you have an integer variable
x
with a value of 5 and you want to perform a division operation that requires a floating-point result, you can typecast x
to a float using the float()
function before performing the calculation.
Float to Integer:
- Typecasting a float to an integer removes the fractional part and truncates the value.
- For example, if you have a float variable
y
with a value of 3.7 and you want to use it as an integer, you can typecast y
to an integer using the int()
function, which will result in a value of 3.
String to Integer/Float:
- Typecasting a string to an integer or float is useful when converting user input or handling numeric values in string format.
- For example, if you have a string variable
num_str
with a value of "10" and you want to perform arithmetic operations on it, you can typecast num_str
to an integer using the int()
function to treat it as a numeric value.
Considerations and Best Practices
While typecasting can be a powerful tool, it must be used carefully to avoid unexpected results or errors. Here are some considerations and best practices when working with typecasting:
Ensure Compatibility:
- Typecasting a variable from one type to another should only be done when the data can be represented in the target type without loss of information.
- For example, converting a large floating-point number to an integer may result in loss of precision.
Handle Possible Data Loss:
- Typecasting between certain data types can lead to data loss or unexpected behavior.
- For example, typecasting a large floating-point number to an integer may result in truncation of the decimal part without rounding.
- It is important to be aware of the possible implications and handle the data accordingly.
Beware of Implicit Typecasting:
- Implicit typecasting can be convenient, but it can also lead to unexpected results if not understood or handled correctly.
- It is recommended to explicitly typecast variables when there is a need for clarity or precision in the data operations.
Follow Language-Specific Guidelines:
- Different programming languages have their own rules and conventions for typecasting.
- It is important to refer to the documentation and guidelines of the specific programming language being used to understand the typecasting rules and best practices.
Related Terms
- Code Injection: The unauthorized insertion of code within a computer program or system.
- Buffer Overflow: A type of security vulnerability where a program writes data to a buffer that exceeds the buffer's boundary, leading to a potential system crash or the execution of harmful code.
By understanding typecasting and its various aspects, programmers can effectively manipulate data types, ensure compatibility, and perform specific operations based on the desired outcomes. It is a fundamental concept in computer programming that plays a crucial role in data manipulation and program execution.